Google provided library of API for Gmail. Gmail API give applications access to the user’s inbox and various elements of the mail box like threads, messages, labels, drafts, history and settings. Using Python or any other modern language application can use the API to read messages, send messages and change message label etc.
A good use of Gmail API is to programmatically clean up the inbox by assigning ‘Trash’ label to old and junk messages. Subsequently, Gmail will permanently delete the message.
This post lists a python script that reads a message, print various information of the message and assigns ‘Trash’ label to it. The script uses oAuth2.0 authentication to access the inbox. Mailbox owner does not have to share the password to run this script. oAuth2.0 allow owner to give permission to the script to access messages without sharing their password.
This post consists of 3 parts.
Part 1 : Python script to access Gmail messages. Complete Part 2 and Part 3 before running code in Part 1
Part 2 : Setup credentials to access Gmail
Part 3 : Create project consent form
Part 1 : Python script to access Gmail messages
Python Code listing
from googleapiclient.discovery import build
from httplib2 import Http
from oauth2client import file, client, tools
scopes = [
'https://www.googleapis.com/auth/gmail.modify'
]
def checkCred():
store = file.Storage('token.json')
creds = store.get()
if not creds or creds.invalid:
flow = client.flow_from_clientsecrets('credentials.json', scopes)
creds = tools.run_flow(flow, store)
service = build('gmail', 'v1', http=creds.authorize(Http()))
return service
def main():
processCnt=1
service = checkCred()
response = service.users().messages().list(userId='me', maxResults=processCnt).execute()
msglist = []
if 'messages' in response:
msglist.extend(response['messages'])
for msg in msglist:
msgid = msg['id'].encode('utf-8')
message = service.users().messages().get(userId='me', id=msgid).execute()
hdr = message['payload']['headers']
print ('msgid = '+msgid)
message = service.users().messages().modify(userId='me', id=msgid,body={"addLabelIds":['TRASH']}).execute()
#print (hdr)
for ea in hdr:
for key, value in ea.items():
print("key: {} | value: {}".format(key, value))
return
if __name__ == '__main__':
main()
- Install packages :
- pip install –user –upgrade google-api-python-client oauth2client. Check for quick start sample
- Set Google scope. Request permission to modify message from the owner:
- scopes = [ ‘https://www.googleapis.com/auth/gmail.modify’]. Gmail provides various scopes that allow different access level. Complete list of scope.
- checkCred() in the code references two files. These files are required to authorize and to get consent from the owner to access their Gmail.
- credentials.json (described in Part 2)
- token.json (created when the script runs the first time)
- processCnt=1 : Process only 1 message. This can be changed as needed.
- for msg in msglist : This loop gets header of the message. Header contains JSON object containing information about the message like From, To, Date, Subject etc. Inside this loop ‘TRASH’ label is assigned to the message.
- for ea in hdr : This loop print all the key/value of header, few key values are shown here:
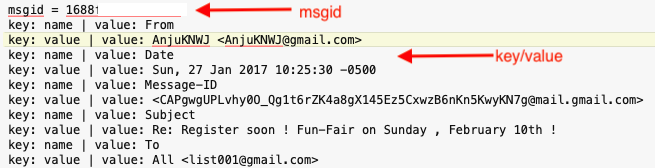
When the script is run for the first time, below consent screen is shown asking for permission. This will create a token.json file in the same folder as the python script. On subsequent runs, this screen will not be shown. The script will continue to use this token as consent from user.
However, if the scope is changed in the script then token.json will need to be re-created. Simply delete the existing token.json before the next run.
After the script has run; simply login to gmail and see that message has moved to ‘trash’ folder.
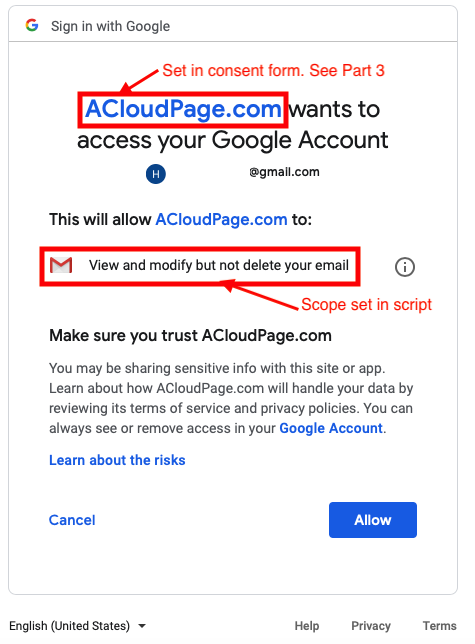
The script can be tweaked to filter out messages that have aged and be scheduled to ‘trash’ them automatically once a day.
Verify with Google API Explorer : You can use API explorer to verify that the msgid (print (msgid)) retrieved by above script in moved to ‘Trash’. Set the values as below on the explorer. Goto Settings and set API key if required prior to executing on the explorer query.
userid=me
id=msgid
format=metadata
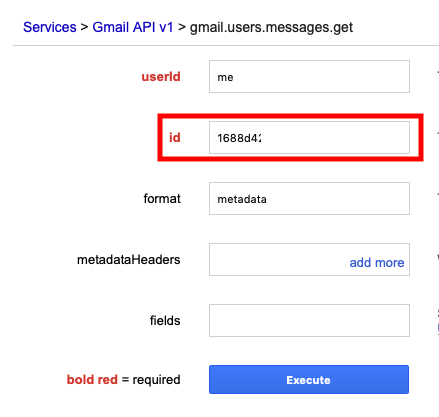
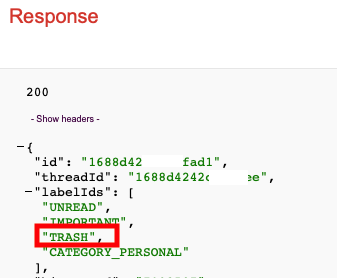
Part 2 : Setup credentials to access Gmail
Step 1 : Log into Google Cloud Platform account and create a new project or use an existing project
Step 2 : Create oAuth2.0 credentials as below
oAuth2.0 credentials allow programatic access to Google service e.g. Gmail. At the end of this step you should be able to download credentials.json file. Save it in the same folder as python script from Part 1.
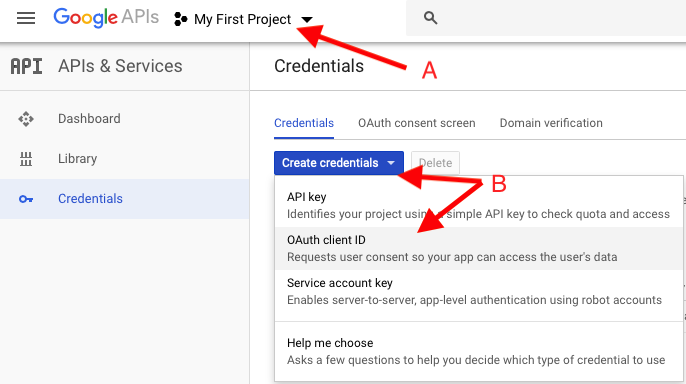
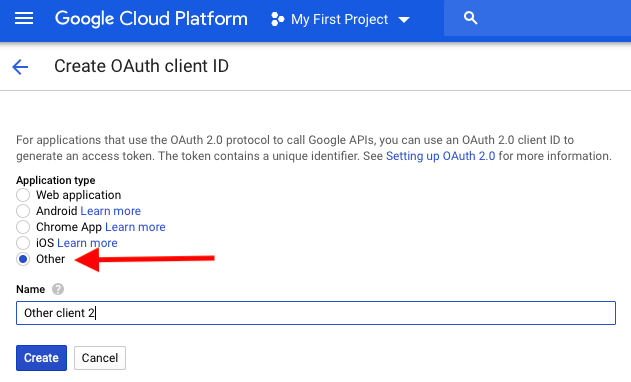
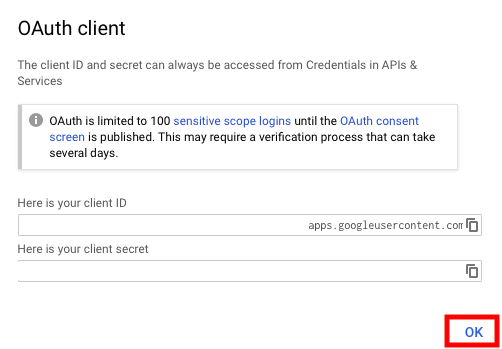

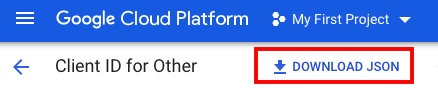
Read on Gmail authorization here
Part 3 : Create project consent form
Create a consent form which will be presented to the owner requesting permission when the python script runs. The consent form tells the user who is requesting access to their Gmail and what kind of data will be access.
Step 1 : You should already by logged into Google Cloud Platform. If not then refer to step 1 in Part 2 above
Step 2 : From “Credentials” on “OAuth consent screen”. Enter Application name, Support email and Save the form. Enter application name which is recognizable by the Gmail owner, to grant access.
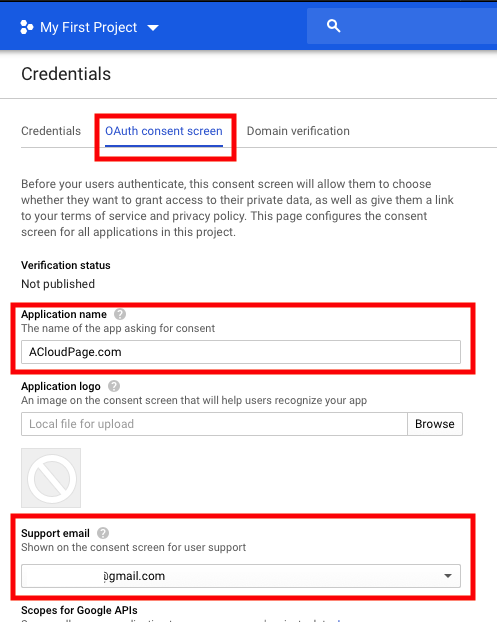
Step 3 : Save. Sample consent form is shown in Part 1.